Tokens are the smallest individual unit in a program. It's also called lexical unit.
Types of token
- Identifiers
- Keywords
- Operators
- Literals
- Punctuators
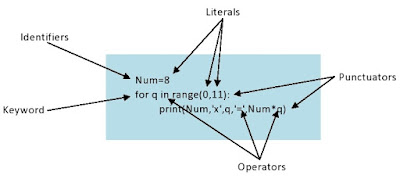
Identifiers
Identifier are used as a name to identify variables, list, dictionaries, classes, objects, variables, functions, etc.
Some rules of making identifier:
• It can be of any length.
• The first character should be a letter or underscore ( _ ).
• Keywords cannot be used as an identifier.
• Digits can be used except in the first character of an identifier.
• No special characters should be used except underscore ( _ ).
For example:
_hello
_Hello
Python1
Keywords
Keywords are reserved words in a programming language which has a special meaning.
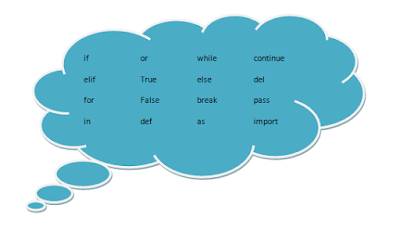
Operators
Operators are the characters that triggers some computation when applied to variables.
- Arithmetic Operators
- Relational Operators
- Membership Operators
- Bitwise Operators
- Identity Operators
- Logical Operators
- Assignment Operators
Literals
Literals are data items that have a fixed value.
- String Literal
- Numeric Literal
- Boolean Literal
- None Literal (Special Literal)
Punctuators
Punctuators are symbols to organise sentence structure, expression, statements and program structure in a programming language.
Skeleton of Python Program
Expression
Expression is any combination of characters and symbols that represent a value.
For example:
a+7, a*5+b+3, etc.
Statement
Statement are used to take some actions or define instructions or functions.
For example:
print ("Hello")
This statement will call print() function.
Function
Function is a group of codes that has its name and it can be executed again by specifying its name.You will get to know more about functions in further chapters.
Blocks
Blocks are the group of statements that is a part of another statement or functions.
For example:
Indentation are used to specify blocks. You cannot use indentation unnecessarily, it will raise an error.
Comments
Comments are just an additional information source, which is read by the programmers, but ignored in execution process. In python, # symbol is used to specify comments.
Dynamic Typing
In python, a variable that points to a value of one type can be use again to points to another value.
For example:
Input Code :
a=5
print(a)
a="hello"
print(a)
Output Code :
5
hello
🙀🙀😻
ReplyDelete